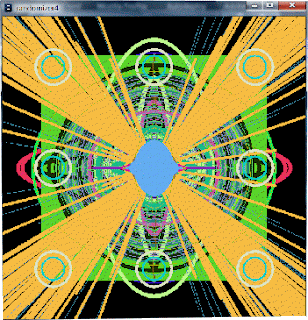
I'm a bit bamboozled about the requirements for the first and second programs. After adding the array function the code is well over 100 lines but I think I could cut down the amount of lines by cramping the code a bit.
Does anyone know if 100 lines is a fixed limit?
1:/*
2: title: Funky Chunk 16
3: description: random pattern generator
4: created: December 2, 2008
5: by: Rosie Wood
6: */
7:// define Global variable values
8://These are constant variables and therefore they are not changed
9://by the program. Setting their value here in one place helps with maimtenance
10:int sizeWindow=600;
11:int centre=sizeWindow/2;
12:int xpos = centre;
13:int ypos = centre;
14:int patternsPerPicture=round(random(2,50));
15:
16:boolean [] recs=new boolean[9];
17:
18://this variable is changed frequently by the application
19:int interval=30;
20:int count;
21:// set the sketch window size and background
22://turn off fill so that patterns underneath can be seen
23://set the rate that the screen refreshes at (found this value was best for this application?)
24:void setup(){
25: size(sizeWindow, sizeWindow);
26: background(0);
27: stroke(204, 102, 0);//colour red
28: noFill();
29: frameRate(.2);
30: draw();
31:}
32:
33:void draw(){
34: //local variables
35: count=0;
36: int design=0;
37: background(0);
38: for(count=0;count<patternsPerPicture;count++){
39: strokeWeight(getSW());//set the strokewright to the number returned by the getSW function
40: noFill();
41: interval=round(random(5,70));//increment by interval in while loops
42: changeColour();
43: design=round(random(1,9)); //a random numver for which design to use in the switch
44: switch(design){
45: case 1:
46: repeatShapes(round(random(3,70)),'2');
47: break;
48: case 2:
49: drawCircles(round(random(20,round(random(1,50))))); //call the drawCircles function
50: break;
51: case 3:
52: repeatShapes(round(random(1,width/2)),'2');//use the drawCircle2 function
53: break;
54: case 4:
55: repeatShapes(width-round(random(2,width/4)),'3');
56: break;
57: case 5:
58: if(round(random(4))==2){
59: doLines();}
60: else{drawSectors();}
61: case 6:
62: repeatShapes(interval,'5');
63: break;
64: case 7:
65: repeatShapes(round(random(1,120)),'2');
66: break;
67: case 8:
68: default:
69: repeatShapes(width-round(random(1,width/2)),'4');//draws unfilled squares
70: }
71: }
72: patternsPerPicture=round(random(2,50));//change number of patterns for next picture
73:}
74:
75:/**function changeColour: to change the stroke colour no parameters no return value*/
76:void changeColour(){
77: stroke(random(255),random(255),random(255));
78:}
79:
80://function draws circles accepts parameter gap and uses it to increment loop untill
81:void drawCircles(int gap){
82: for(int n=0;n<round(random(width/2,width));n+=gap){
83: drawCircle(n);
84: }
85:}
86:
87:/**no parameters, no return valur*/
88:void drawCircle2(int diameter){
89: ellipse(xpos, ypos, diameter-random(5,diameter), diameter-random(5,diameter));
90:}
91:
92://accepts 1 parameter
93:void drawCircle3(int diameter){
94: ellipse(xpos, ypos, (diameter*random(5,diameter))/(random(5,diameter)*19), (diameter*random(5,diameter))/(random(5,diameter)*19) );
95:}
96:
97:void drawSquares(int diameter){
98: int r= round(random(1,4));
99: rectMode(RADIUS);
100: //if/if else/else block
101: if(r==1){
102: noStroke();
103: rectMode(CENTER);
104: }
105: else if(r==2){
106: diameter=diameter/20;
107: }
108: else if(r==3){
109: changeColour();
110: }
111: else{
112: diameter=diameter/20;
113: stroke(round(random(255)),round(random(255)),round(random(255)),round(random(255)));
114: }
115:
116: rect(xpos,ypos,diameter,diameter);
117:}
118:
119://returns integer valur for stroke weight depending on framecount
120:int getSW(){
121: int fc=round(random(frameRate*500)/5);
122: return fc%10;
123: }
124:
125:void repeatShapes(int gap, char c){
126: char shape=c;
127: int interval=gap;
128: while(interval<width){//while intervel is less than the width of the window
129: if(shape=='1')drawCircles(interval);
130: else if(shape=='2'){
131: drawCircle2(interval);
132: }//call function drawCircle2 with a random parameter
133: else if(shape=='3'){
134: drawCircle3(interval);
135: }
136: else if(shape=='4'){
137: int r=round(random(1,5));
138: if(r==1){
139: noStroke();
140: changeColour();
141: fill(random(255),random(255),random(255));}
142: drawSquares(round(interval));
143: }
144: else{
145: int r=round(random(1,5));
146: if(r==1){
147: noStroke();
148: fill(random(255),random(255),random(255));}
149: drawCircle2(interval/4); }
150: interval+=gap;}}
151:
152:void doLines(){
153: strokeWeight(getSW());
154: for(int b=1;b<width;b=b+round(random(1,20))){
155: int down=round(random(width/2));
156: int across=round(random(width/2));
157: line(xpos,ypos,across-b,down-b);
158: line(xpos,ypos,across+b+xpos,down-b);
159: line(xpos,ypos,across-b,width-down+b);
160: line(xpos,ypos,across+b+xpos,width-down+b);}}
161:
162:void drawCircle(int diameter){
163: ellipse(xpos, ypos, diameter-interval, diameter);
164: ellipse(xpos, ypos, diameter, diameter-interval);
165: ellipse(xpos, ypos, diameter, diameter);
166:}
167:
168:void resetRecs(){
169: for(int r=0;r<9;r++){
170: recs[r]=false;}}
171:
172:
173:void drawSectors(){
174: resetRecs();
175: int [] midxy={(width/3)/2, (width/3)/2+width/3, (width/3)/2+width/3*2 };
176:
177: //decide on groups to use
178: int corners=round(random(0,1));
179: if(corners==1){
180: recs[0]=recs[2]=recs[6]=recs[8]=true;
181: }
182: int sides=round(random(0,1));
183: if(sides==1){
184: recs[1]=recs[3]=recs[5]=recs[7]=true;
185: }
186: int centre=round(random(0,1));
187: if(centre==1){
188: recs[4]=true;
189: }
190: for(int r=0;r<9;r++){
191: println("num"+r+"rec? "+recs[r]);
192: }
193: rectMode(CENTER);
194: ellipseMode(CENTER);
195: int f=round(random(1,5));
196: if(f==1){
197: fill(random(255),random(255),random(255));}
198: else{
199: getSW();
200: changeColour();
201: }
202: int d=round(random(width/3));
203: int sType=round(random(2));
204: for(int n=0;n<9;n++){
205: if(recs[n]==true){
206: int mod=n%3;
207: int down=0;
208: if(n>5)down=2;
209: else if (n>2){down=1;}
210:
211: if(sType==1){
212: rect(midxy[mod], midxy[down], d, d); }
213: else{
214: ellipse(midxy[mod], midxy[down], d, d);}
215: println("mod: "+mod);
216: println("down: "+down);
217: println("across: "+midxy[mod]);
218: println("down: "+midxy[down]);
219:
220: }
221: }
222:
223:
224:}
No comments:
Post a Comment